How to create an Android Launcher App
Posted on July 26, 2017 • 3 minutes • 459 words
Table of contents
What is a Launcher App?
A Launcher app is the app that comes up when you press the home button or when you start your Android device.The main purpose of this app is to start other apps, but it can also host widgets from other apps.
I created a small app you can use to learn how to create a launcher app. You can find the Github project here
How does it look like?
My demo app has two fragments.
Homescreen:
The homescreen with a list of the installed apps. Here you can start other apps.
Widgetscreen:
Here is just a button that will open the widget picker. The selected widget will be displayed below in the FrameLayout.
How to start?
To get your app registered by the Android OS as an launcher app, it takes nothing more than to add these intent filters to your AndroidManifest.xml:<intent-filter>
<category android:name="android.intent.category.HOME" />
<category android:name="android.intent.category.LAUNCHER"/>
<category android:name="android.intent.category.DEFAULT" />
</intent-filter>
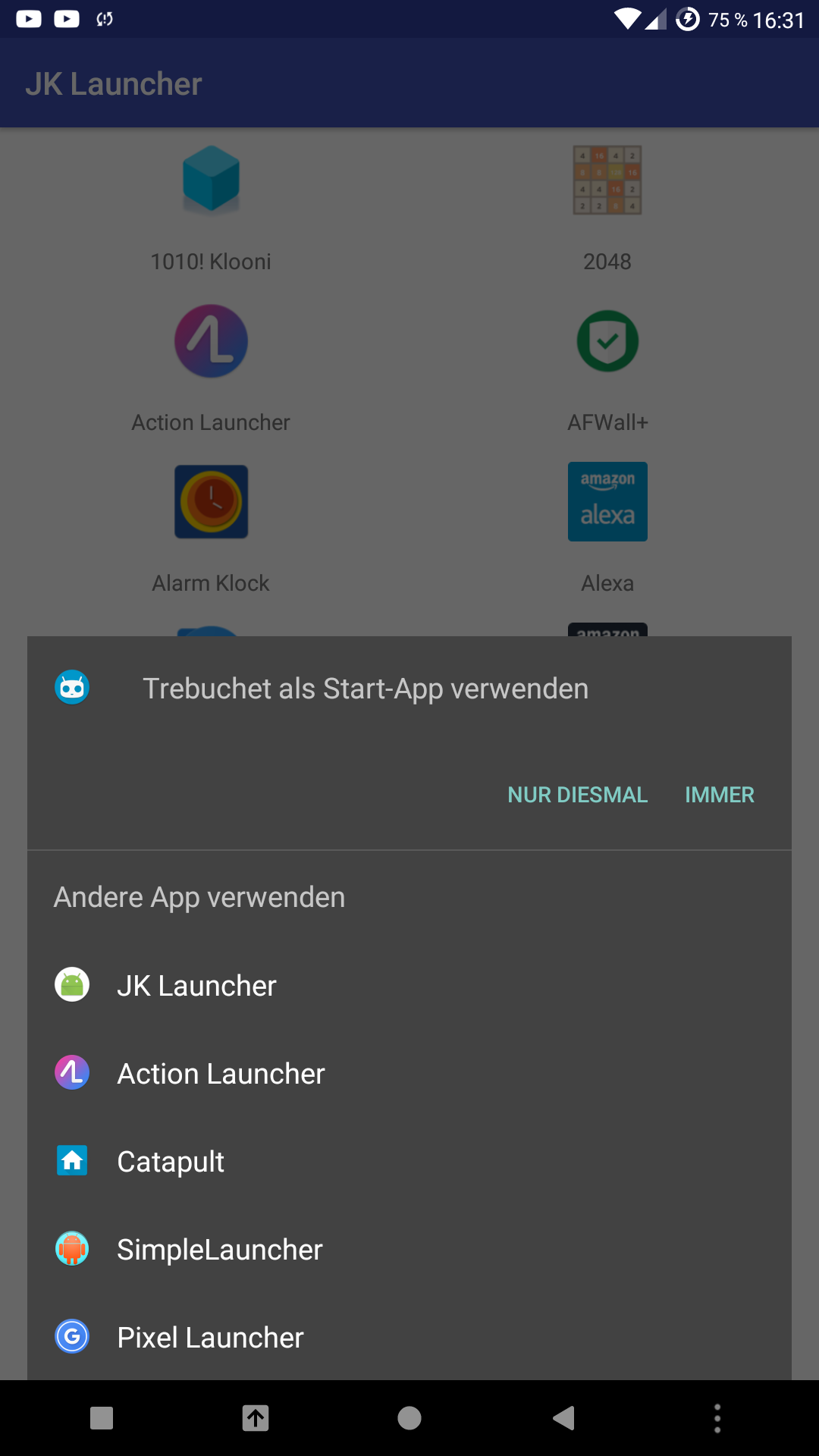
After adding the intent filters, you can choose your app as an standard launcher.
Homescreen
How to get a list of the installed apps?
To get the list of the installed apps you need to use the PackageManager class.
public static List<ApplicationInfo> getAllInstalledApplications(Context context) {
List<ApplicationInfo> installedApps =
context.getPackageManager().getInstalledApplications(PackageManager.PERMISSION_GRANTED);
List<ApplicationInfo> launchableInstalledApps = new ArrayList<ApplicationInfo>();
for (int i = 0; i < installedApps.size(); i++) {
if (context.getPackageManager().getLaunchIntentForPackage(installedApps.get(i).packageName)
!= null) {
launchableInstalledApps.add(installedApps.get(i));
}
}
return launchableInstalledApps;
}
This list will be passed to a RecyclerView. The PackageManager provides methodes to get the needed information about a ApplicationInfo.
AppName: getPackageManager().getApplicationLabel(ApplicationInfo info)
AppIcon: getPackageManager().getApplicationIcon(ApplicationInfo info)
AppIntent: getPackageManager().getLaunchIntentForPackage(ApplicationInfo info)
Widgetscreen
Pick a widget
void selectWidget() {
int appWidgetId = this.mAppWidgetHost.allocateAppWidgetId();
Intent pickIntent = new Intent(AppWidgetManager.ACTION_APPWIDGET_PICK);
pickIntent.putExtra(AppWidgetManager.EXTRA_APPWIDGET_ID, appWidgetId);
startActivityForResult(pickIntent, REQUEST_PICK_APPWIDGET);
}
Start an intent with the AppWidgetManager to open the Widget Picker.
Receive the result
@Override public void onActivityResult(int requestCode, int resultCode, Intent data) {
if (resultCode == RESULT_OK) {
if (requestCode == REQUEST_PICK_APPWIDGET) {
configureWidget(data);
} else if (requestCode == REQUEST_CREATE_APPWIDGET) {
createWidget(data);
}
} else if (resultCode == RESULT_CANCELED && data != null) {
int appWidgetId = data.getIntExtra(AppWidgetManager.EXTRA_APPWIDGET_ID, -1);
if (appWidgetId != -1) {
mAppWidgetHost.deleteAppWidgetId(appWidgetId);
}
}
}
In onActivityResult you check if the user picked a widget or pressed the back button.
Show the widget
public void createWidget(Intent data) {
Bundle extras = data.getExtras();
int appWidgetId = extras.getInt(AppWidgetManager.EXTRA_APPWIDGET_ID, -1);
AppWidgetProviderInfo appWidgetInfo = mAppWidgetManager.getAppWidgetInfo(appWidgetId);
AppWidgetHostView hostView =
mAppWidgetHost.createView(getContext().getApplicationContext(), appWidgetId, appWidgetInfo);
hostView.setAppWidget(appWidgetId, appWidgetInfo);
frameLayout.removeAllViews();
frameLayout.addView(hostView);
Log.i(TAG, "The widget size is: " + appWidgetInfo.minWidth + "*" + appWidgetInfo.minHeight);
}
The Widget is inside a AppWidgetHostView. Add this view to a layout.
That's all it takes to create a very simple launcher app. I hope the information is useful for you.